The article discusses how to document C# code with XML comments in Visual Studio. Also, it explains why it is useful and demonstrates the use of the different XML tags for documentation.
If you are newbie in C#, visit the links: C# Tutorial and XML documentation comments to start.
What are XML Comments and why are they useful?
You can create XML documentation from a C# project. Also, the XML comments are useful if you use IntelliCode and Assisted IntelliSense.
To create XML documentation you should enable the field Generate a file containing API documentation
.:
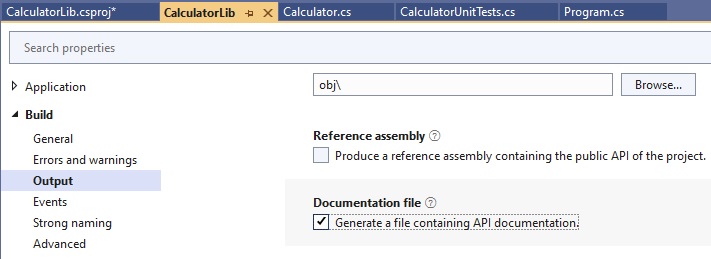
Let us to write little application and demonstrate XML comments work. Create a simple class Calculator in C# which calculates addition and subtraction of the numbers:
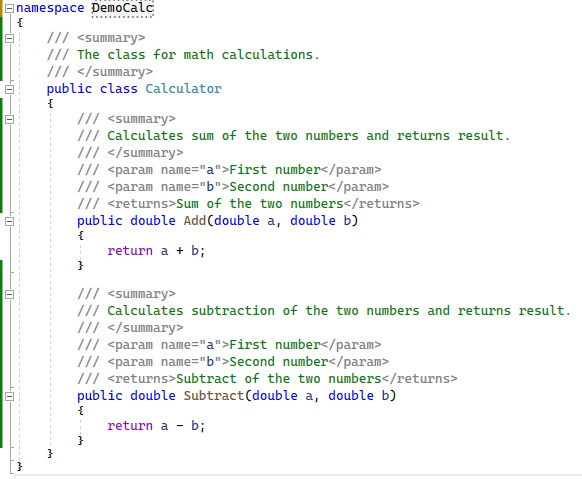
Take note that the class itself has XML comment as well as class methods have their own XML comments.
Now, let us to consume this class by some code and move the mouse cursor to class name:
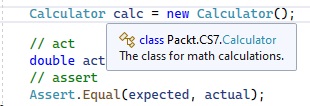
Good news! IntelliCode algorithm shows us our XML comments as code tips.
Now, it’s time to try same for the class methods:

And success again! you see method tips and it helps you to remember what this method does.
Essential XML comment tags
summary
The summary
tag adds brief information about what type of member is (class, method):
/// <summary>
/// The main Math class.
/// Contains all methods for performing basic math functions.
/// </summary>
remarks
The remarks
tag supplements the information about types or members that the summary
tag provides:
// Adds two integers and returns the result
/// <summary>
/// Adds two integers and returns the result.
/// </summary>
/// <returns>
/// The sum of two integers.
/// </returns>
returns
The returns
tag describes the return value of a method declaration:
/// Adds two integers and returns the result
/// <summary>
/// Adds two integers and returns the result.
/// </summary>
/// <returns>
/// The sum of two integers.
/// </returns>
value
The value
tag is similar to the returns
tag, except that you use it for properties:
public class Math
{
/// <value>Gets the value of PI.</value>
public static double PI { get; }
}
example
You use the example
tag to include an example in your XML documentation. This involves using the child code
tag:
/// <example>
/// <code>
/// int c = Math.Add(4, 5);
/// if (c > 10)
/// {
/// Console.WriteLine(c);
/// }
/// </code>
/// </example>
para
You use the para
tag to format the content within its parent tag. para
is usually used inside a tag, such as: summary
, remarks
, or returns
to divide text into paragraphs:
<remarks>
/// <para>This class can add, subtract, multiply and divide.</para>
/// <para>These operations can be performed on both integers and doubles.</para>
/// </remarks>
c
You use the tag to mark part of text as code. It’s like the tag code
but inline. It’s useful when you want to show a quick code example as part of a tag’s content:
/// The main <c>Math</c> class.
/// Contains all methods for performing basic math functions.
/// </summary>
exception
By using the exception
tag, you let your developers know that a method can throw specific exceptions:
/// <exception cref="System.OverflowException">Thrown when one parameter is max
/// and the other is greater than 0.</exception>
see
The see
tag lets you create a clickable link to a documentation page for another code element:
/// See <see cref="Math.Add(double, double)"/> to add doubles.
seealso
You use the seealso
tag in the same way you do the see
tag. The only difference is that its content is typically placed in a “See Also” section:
/// See <see cref="Math.Add(double, double)"/> to add doubles.
/// <seealso cref="Math.Subtract(int, int)"/>
/// <seealso cref="Math.Multiply(int, int)"/>
param
You use the param
tag to describe a method’s parameters:
/// <param name="a">A double precision number.</param>
/// <param name="b">A double precision number.</param>
typeparam
You use the typeparam
tag just like the param
tag but for generic type or method declarations to describe a generic parameter. An example:
/// <typeparam name="T">A type that inherits from the IComparable interface.</typeparam>
public static bool GreaterThan<T>(T a, T b) where T : IComparable
{
return a.CompareTo(b) > 0;
}
paramref
Sometimes you might be in the middle of describing what a method does in what could be a summary
tag, and you might want to make a reference to a parameter. See an example:
/// <summary>
/// Adds two doubles <paramref name="a"/> and <paramref name="b"/> and returns the result.
/// </summary>
typeparamref
You use the typeparamref
tag just like the tag but for generic type or method declarations to describe a generic parameter:
/// <typeparam name="T">A type that inherits from the IComparable interface.</typeparam>
public static bool GreaterThan<T>(T a, T b) where T : IComparable
{
return a.CompareTo(b) > 0;
}
list
You use the list
tag to format documentation information as an ordered list, an unordered list, or a table. Examine this example:
/// <summary>
/// The main <c>Math</c> class.
/// Contains all methods for performing basic math functions.
/// <list type="bullet">
/// <item>
/// <term>Add</term>
/// <description>Addition Operation</description>
/// </item>
/// <item>
/// <term>Subtract</term>
/// <description>Subtraction Operation</description>
/// </item>
/// <item>
/// <term>Multiply</term>
/// <description>Multiplication Operation</description>
/// </item>
/// <item>
/// <term>Divide</term>
/// <description>Division Operation</description>
/// </item>
/// </list>
/// </summary>
Was this helpful?
0 / 0